Patterns in Java
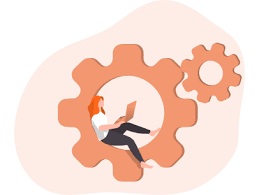
Nested Loops
This article displays the logic of pattern programs in java using nested loops. The program includes printing a series of patterns. A developer should have a good understanding of the programming language and the syntax of the language. They must also have a strong logic and algorithm-building ability.
Often the developer is under pressure to develop an algorithm quickly and efficiently using a programming language for a prescribed problem. The stress is in the creation of the steps of the program that can easily resolve the problem without throwing any logical or syntactical errors.
Loops or iterations can be used in different ways to formulate the algorithm of patterns in java using loops or nested loops. Iterative constructs allow a group of statements to get executed repeatedly till a test expression is evaluated to be true is called a loop.
The statements in the block may be executed any number of times depending upon the user’s input. A loop that continues forever is called an infinite loop and is considered an error in the development of an algorithm because it can easily hang the system.
Every loop has certain elements that control the execution of the loop. The Initialization Expression, the variable that controls the execution is called the Initialization. The initialization may occur inside or outside the loop. The second expression is the test expression that decides whether the statements inside the loop will be executed or not.
Till the test expression evaluates to true, the loop body gets executed, or else the loop gets terminated. The third expression is the update expression keeping a count of the number of iterations. The update expression is usually made to be executed before the beginning of the next iteration.
When a loop is placed within a loop, then that is called a nested loop. The usage of nested loops depends upon the program algorithm based on the output required to be generated. In nested loops, it must be remembered that the control is transferred to the outer loop only when the inner loop completes its iterations unless the developer breaks out of the loop.
Displaying patterns in java employs the usage of nested loops. The process of developing the algorithm to use nested loops in a precise manner within a time-boxed period is looked at by the interviewers. Hence pattern printing programs are a great choice for interviews. Learning to display pattern programming involves deep knowledge of how loops behave. Here is a list of top pattern programs using java that are important for students, developers, and programmers.
Pattern 1
Write a programme to print the pattern:
1
2 3
4 5 6
7 8 9 10
11 12 13 14 15
/**
* Prints the numbers pattern 1
* Prasun Bhattacharya
* 06-Mar-2023
*/
public class Pattern1
{
public static void main(String args[])
{
int num = 1;
for (int i = 1; i <= 5; i++) // Outer loop
{
for (int j = 1; j <= i; j++) // Inner loop
{
System.out.print(num++ + "\t"); // Display the numbers
}
System.out.println(); // Next line
}
}
}
Pattern 2
Write a programme to print the pattern:
1 * * * *
2 2 * * *
3 3 3 * *
4 4 4 4 *
5 5 5 5 5
/**
* Prints the numbers pattern 2
* Prasun Bhattacharya
* 06-Mar-2023
*/
public class Pattern2
{
public static void main(String args[])
{
for (int i = 1; i <= 5; i++) // Outer loop
{
for (int j = 1; j <= i; j++) // Inner loop
{
System.out.print(i + "\t"); // Display the numbers
}
for (int k = 1; k <= 5 - i; k++) // Inner loop to print stars
{
System.out.print('*' + "\t"); // Display the Stars
}
System.out.println(); // Next line
}
}
}
Pattern 3
Write a programme to print the pattern:
A B C D E
A B C D
A B C
A B
A
/**
* Prints the numbers pattern 3
* Prasun Bhattacharya
* 06-Mar-2023
*/
public class Pattern3
{
public static void main(String args[])
{
for (int i = 69; i >= 65; i--) // Outer loop ASCII value of characters
{
for (int j = 65; j <= i; j++) // Inner loop ASCII value of characters
{
System.out.print((char) j + "\t"); // Display the characters
}
System.out.println(); // Next line
}
}
}
Pattern 4
Write a programme to print the pattern:
5 4 3 2 1
4 3 2 1
3 2 1
2 1
1
/**
* Prints the numbers pattern 4
* Prasun Bhattacharya
* 06-Mar-2023
*/
public class Pattern4
{
public static void main(String args[])
{
for (int i = 5; i >= 1; i--) // Outer loop
{
for (int j = i; j >=1; j--) // Inner loop
{
System.out.print( j + "\t"); // Display the numbers
}
System.out.println(); // Next line
}
}
}
Pattern 5
Write a programme to print the pattern:
* * * * 5
* * * 4
* * 3
* 2
1
/**
* Prints the numbers pattern 5
* Prasun Bhattacharya
* 06-Mar-2023
*/
public class Pattern5
{
public static void main(String args[])
{
for (int i = 5; i >= 1; i--) // Outer loop
{
for (int j = i-1; j >=1; j--) // Inner loop
{
System.out.print( '*' + " "); // Display the stars
}
System.out.println(i);
System.out.println(); // Next line
}
}
}
Pattern 6
Write a programme to print the pattern:
* * * * *
* * * *
* * *
* *
*
/**
* Prints the numbers pattern 6
* Prasun Bhattacharya
* 06-Mar-2023
*/
public class Pattern6
{
public static void main(String args[])
{
for (int i = 5; i >= 1; i--) // Outer loop
{
for (int j = 1; j <=i; j++) // Inner loop
{
System.out.print( '*' + " "); // Display the stars
}
System.out.println(); // Next line
}
}
}
Pattern 7
Write a programme to print the pattern:
*
* *
* * *
* * * *
* * * * *
/**
* Prints the numbers pattern 7
* Prasun Bhattacharya
* 06-Mar-2023
*/
public class Pattern7
{
public static void main(String args[])
{
for (int i = 1; i <= 5; i++) // Outer loop
{
for (int j = 1; j <=i; j++) // Inner loop
{
System.out.print( '*' + " "); // Display the stars
}
System.out.println(); // Next line
}
}
}
Pattern 8
Write a programme to print the pattern:
1
2 2
3 3 3
4 4 4 4
5 5 5 5 5
/**
* Prints the numbers pattern 8
* Prasun Bhattacharya
* 06-Mar-2023
*/
public class Pattern8
{
public static void main(String args[])
{
for (int i = 1; i <= 5; i++) // Outer loop
{
for (int j = 1; j <=i; j++) // Inner loop
{
System.out.print( i + " "); // Display the numbers
}
System.out.println(); // Next line
}
}
}
Pattern 9
Write a programme to print the pattern:
*
* *
* * *
* * * *
* * * * *
/**
* Prints the numbers pattern 9
* Prasun Bhattacharya
* 06-Mar-2023
*/
public class Pattern9
{
public static void main(String args[])
{
for (int i = 1; i <= 5; i++) // Outer loop
{
for (int j = 5-1; j >1; j--) // Inner loop
{
System.out.print(" "); // Display space
}
for (int j = 0; j<= i; j++)
{
System.out.print(" "); // Display stars
}
System.out.println(); // Next line
}
}
}
Pattern 10
Write a programme to print the pattern:
#
* *
###
* * * *
#####
/**
* Prints the numbers pattern 10
* Prasun Bhattacharya
* 06-Mar-2023
*/
public class Pattern10
{
public static void main(String args[])
{
for (int i = 1; i <= 5; i++) // Outer loop
{
for (int j = 1; j<=1; j++) // Inner loop
{
if (i%2 == 0)
{
System.out.print("* "); // Display star
}
else
{
System.out.print("# "); // Display hash
}
}
System.out.println(); // Next line
}
}
}
Pattern 11
Write a programme to print the pattern:
*
* *
* * *
* * * *
* * * * *
* * * * * *
* * * * * * *
* * * * * * * *
* * * * * * * * *
* * * * * * * *
* * * * * * *
* * * * * *
* * * * *
* * * *
* * *
* *
*
/**
* Prints the numbers pattern 11
* Prasun Bhattacharya
* 06-Mar-2023
*/
public class Pattern11
{
public static void main(String args[])
{
for (int i = 0; i <= 9-1; i++) // Outer loop
{
for (int j = 0; j<=i; j++) // Inner loop
{
System.out.print("*" + " "); // Display star
}
System.out.println(); // Next line
}
for (int i = 9-1; i >= 0; i--) // Outer loop
{
for (int j = 0; j<=i-1; j++) // Inner loop
{
System.out.print("*" + " "); // Display star
}
System.out.println(); // Next line
}
}
}
Pattern 12
Write a programme to print the pattern:
*
* * *
* * * * *
* * * * * * *
* * * * * * * * * *
* * * * * * * * * * *
* * * * * * * * *
* * * * * * *
* * * * *
* * *
*
/**
* Prints the numbers pattern 12
* Prasun Bhattacharya
* 06-Mar-2023
*/
public class Pattern12
{
public static void main(String args[])
{
int blankspace=9-1;
for (int x = 1; x <= 9; x++) // Outer loop
{
for (int y = 1; y<=blankspace; y++) // Inner loop
{
System.out.print(" "); // Display space
}
blankspace -- ;
for (int y = 1; y<=2 * x - 1; y++) // Inner loop
{
System.out.print("*"); // Display star
}
System.out.println();
}
blankspace = 1;
for (int x = 1; x >= 9-1; x++) // Outer loop
{
for (int y = 1; y<= blankspace; y++) // Inner loop
{
System.out.print(" "); // Display space
}
for (int y = 1; y<=2 * (9 - x) - 1; y++) // Inner loop
{
System.out.print("*"); // Display star
}
System.out.println(); // Next line
}
}
}




